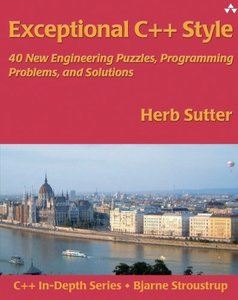
Exceptional C++ Style : 40 New Engineering Puzzles, Programming Problems, and Solutions
內容描述
Table of
Contents:
Preface.
GENERIC PROGRAMMING AND THE C++ STANDARD
LIBRARY.
- Uses and Abuses of vector.
- The String Formatters of Manor Farm, Part 1:
sprintf. - The String Formatters of Manor Farm, Part 2:
Standard (or Blindingly Elegant) Alternatives. - Standard Library Member Functions.
- Flavors of Genericity, Part 1: Covering the
Basis [sic]. - Flavors of Genericity, Part 2: Generic
Enough? - Why Not Specialize Function
Templates? - Befriending Templates.
- Export Restrictions, Part 1:
Fundamentals. - Export Restrictions, Part 2: Interactions,
Usability Issues, and Guidelines.
EXCEPTION SAFETY ISSUES AND
TECHNIQUES. - Try and Catch Me.
- Exception Safety: Is It Worth It?
- A Pragmatic Look at Exception
Specifications.
CLASS DESIGN,
INHERITANCE, AND POLYMORPHISM. - Order, Order!
- Uses and Abuses of Access Rights.
- (Mostly) Private.
- Encapsulation.
- Virtuality.
- Enforcing Rules for Derived
Classes.
MEMORY AND RESOURCE
MANAGEMENT. - Containers in Memory, Part 1: Levels of
Memory Management. - Containers in Memory, Part 2: How Big Is It
Really? - To new, Perchance to throw,
Part 1: The Many Faces of new. - To new, Perchance to throw,
Part 2: Pragmatic Issues in Memory Management.
OPTIMIZATION AND
EFFICIENCY. - Constant Optimization?
- inline Redux.
- Data Formats and Efficiency, Part 1: When
Compression Is the Name of the Game. - Data Formats and Efficiency, Part 2: (Even
Less) Bit-Twiddling.
TRAPS, PITFALLS, AND
PUZZLERS. - Keywords That Aren't (or, Comments by Another
Name). - Is It Initialization?
- double or Nothing.
- Amok Code.
- Slight Typos? Graphic Language and Other
Curiosities. - Operators, Operators
Everywhere.
STYLE CASE STUDIES. - Index Tables.
- Generic Callbacks.
- Construction Unions.
- Monoliths "Unstrung," Part 1: A Look at
std::string. - Monoliths "Unstrung," Part 2: Refactoring
std::string. - Monoliths "Unstrung," Part 3:
std::string Diminishing. - Monoliths "Unstrung," Part 4:
std::string Redux.
Bibliography.
Index.